Laravel queues provide a powerful and efficient way to offload time-consuming tasks, allowing your application to stay responsive and handle a large number of requests.
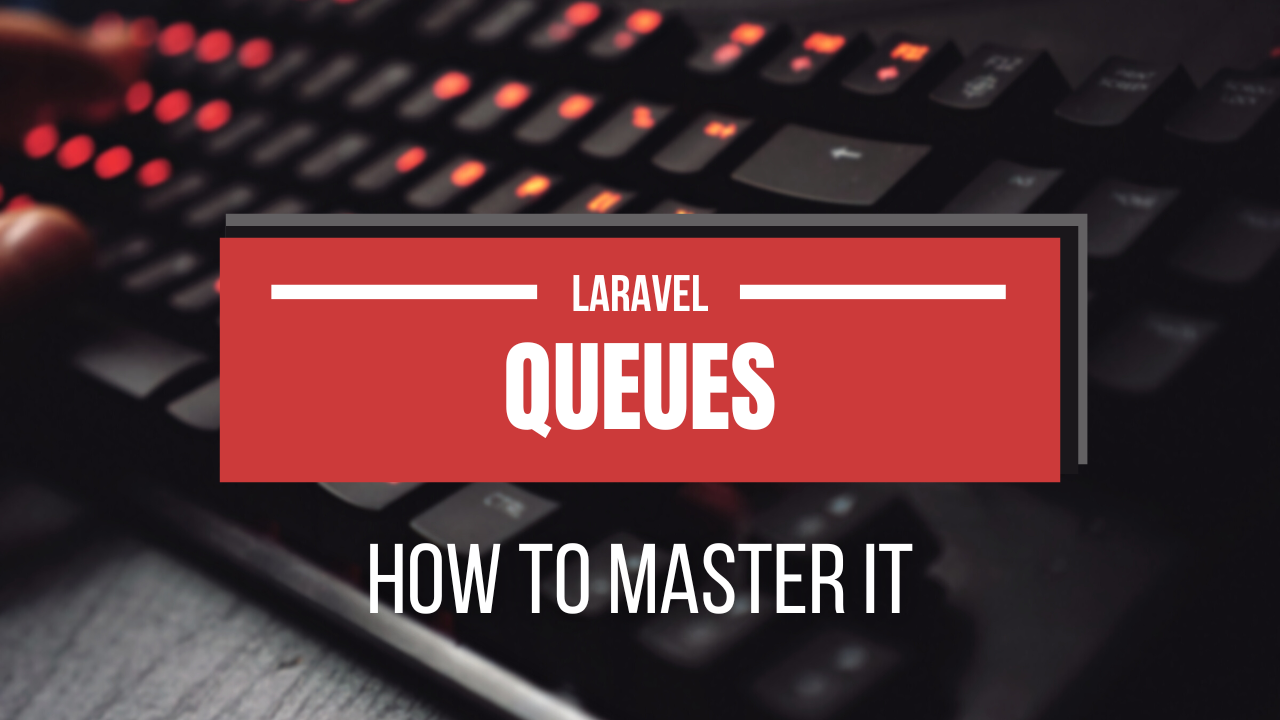
Laravel queues provide a powerful and efficient way to offload time-consuming tasks, allowing your application to stay responsive and handle a large number of requests. In this article, we'll explore the inner workings of Laravel queues and learn how to implement them effectively in your application. We'll also cover the use of queue jobs and queue mail, along with differences between cron and queue in Laravel. By the end of this comprehensive guide, you'll have a solid understanding of Laravel queues and their benefits.
Laravel queues work by allowing you to defer the execution of a time-consuming task to a later time, while your application continues processing other requests. Queues serve as a background task manager that runs these deferred tasks in the background as resources become available. Laravel uses a "first-in, first-out" approach, which means that tasks are executed in the order they are added to the queue.
Implementing queues in Laravel involves the following steps:
- Configuration: Open the
config/queue.php
file and configure your desired queue driver (e.g., Redis, SQS, Beanstalkd, or database). - Job creation: Create a job class using the
php artisan make:job
command. - Job dispatch: Dispatch your job using the
dispatch
method. - Worker management: Start your queue worker by running the
php artisan queue:work
command in your terminal.
Queue jobs in Laravel are represented by job classes that contain the logic required to perform a specific task. To create a job class, run the following command:
php artisan make:job MyJob
This command creates a new job class within the app/Jobs
directory. You can define your task logic within the handle
method of the job class. To dispatch a job, simply call the dispatch
method:
MyJob::dispatch();
Laravel allows you to send emails using queued jobs to avoid delays in your application. To send a queued email, you need to create a Mailable class:
php artisan make:mail MyMail
After customizing your email within the Mailable class, you can send it using the queue
method instead of the send
method:
Mail::to('[email protected]')->queue(new MyMail());
Cron jobs are scheduled tasks that run at fixed intervals, while Laravel queues are background tasks that run as resources become available. Cron jobs are suitable for tasks that need to be executed periodically, such as daily reports or database backups. Queues, on the other hand, are ideal for tasks that can be deferred and executed in the background, such as sending emails or processing images.
Laravel provides a simple way to monitor and manage failed jobs through the failed_jobs
table. To create this table, you need to run the following command:
php artisan queue:failed-table
php artisan migrate
When a job fails, it will be logged in the failed_jobs
table. You can view the list of failed jobs by running the following command:
php artisan queue:failed
If you're using Redis as your queue driver, you can utilize Laravel Horizon for monitoring and managing your queues. Laravel Horizon is an open-source dashboard and monitoring solution designed specifically for Laravel and Redis queues. To install Laravel Horizon, follow these steps:
- Install Horizon via Composer:
composer require laravel/horizon
-
Publish Horizon's assets:
php artisan horizon:install
- Start Horizon by running:
php artisan horizon
Once installed, you can access the Horizon dashboard at /horizon
within your application's URL. The dashboard provides a comprehensive overview of your queues, including job throughput, job failures, retry statistics, and more.
Another approach to monitor the queue status is to implement custom logging within your job classes. You can use Laravel's built-in logging functionality to record information about the progress of your jobs. For example, you can add log entries within the handle
method of your job class:
public function handle()
{
Log::info('Job started: ' . $this->job->getJobId());
// Job processing logic here
Log::info('Job completed: ' . $this->job->getJobId());
}
By monitoring log files, you can keep track of the status of your queued jobs.
Laravel queues offer a powerful and efficient way to manage time-consuming tasks in your application. By leveraging queue jobs and queue mail, you can offload resource-intensive tasks to the background, ensuring that your application remains responsive and performs well under heavy loads. Understanding the differences between cron jobs and queues in Laravel will help you make informed decisions about when to use each approach. With this comprehensive guide, you'll be well-equipped to harness.