In the world of web development, validating user inputs is a crucial aspect of ensuring data integrity, security, and overall functionality. Laravel, a powerful and elegant PHP framework, makes validation a breeze with its built-in features.
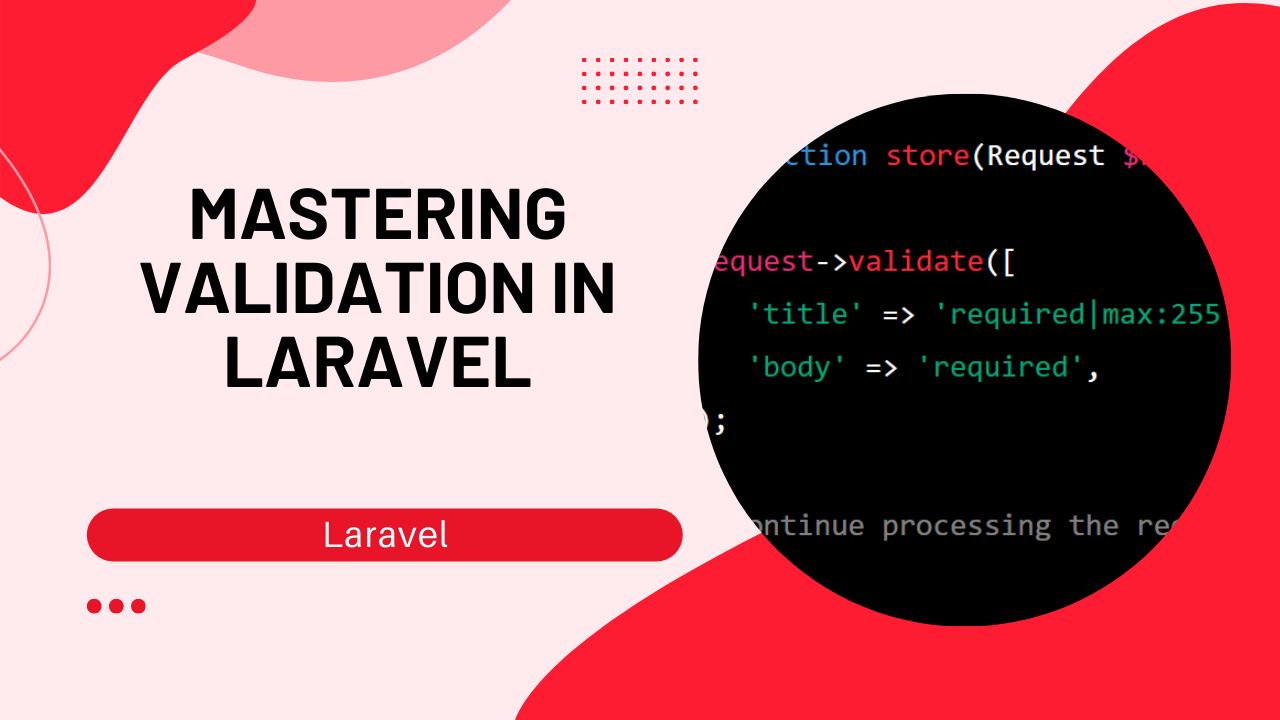
In the world of web development, validating user inputs is a crucial aspect of ensuring data integrity, security, and overall functionality. Laravel, a powerful and elegant PHP framework, makes validation a breeze with its built-in features. In this article, we'll explore various validation techniques in Laravel, including using validation in different versions of Laravel, API validation, and creating custom validation rules.
- Applying Validation in a Laravel Form: To apply validation in a Laravel form, you can use the
validate()
method in the controller. This method accepts an array of validation rules as a parameter. Here's an example:
public function store(Request $request)
{
$request->validate([
'title' => 'required|max:255',
'body' => 'required',
]);
// Continue processing the request...
}
- Using Validation in Laravel 8: Laravel 8 introduced the concept of "Form Request" classes. To use validation in Laravel 8, create a form request class using the command
php artisan make:request MyFormRequest
. Then, define your rules in therules()
method:
public function rules()
{
return [
'title' => 'required|max:255',
'body' => 'required',
];
}
Inject the form request into your controller's method:
public function store(MyFormRequest $request)
{
// Continue processing the request...
}
-
Adding Validation in Laravel: As discussed above, you can add validation using the
validate()
method or by creating a form request class. -
Using Validation Errors in Laravel: Laravel automatically handles validation errors and redirects the user back to the previous page with an error message bag. To display the errors, add the following code in your Blade template:
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
-
Laravel Validation for API: For API validation, you can use the
validate()
method in your controller. If validation fails, Laravel will return a JSON response containing the error messages. Alternatively, you can create a custom response using a form request class and overriding thefailedValidation()
method. -
Applying Validation in Laravel 9 & 10: The process of applying validation in Laravel 9 & 10 is the same as Laravel 8, using form request classes and the
validate()
method. -
Validating Login in Laravel: To validate a user's login, use the built-in authentication scaffold provided by Laravel, or customize the login validation by modifying the
LoginController
. You can utilize Laravel's built-in validation rules for email and password, such as 'required', 'email', and 'min:length'. -
Setting Up Validation: To set up validation in Laravel, use either the
validate()
method or create a form request class, as explained in earlier sections. -
Creating a Validation Rule in Laravel: To create a custom validation rule, run
php artisan make:rule MyRule
. Define the validation logic in thepasses()
method and the error message in themessage()
method. -
Validating Exact Words in Laravel: To validate exact words, use the
in
rule:
'color' => 'required|in:red,green,blue',
In conclusion, understanding and implementing Laravel's built-in validation features is crucial for creating secure, functional, and user-friendly web applications. By applying validation rules in forms, leveraging Form Request classes, and handling validation errors effectively, you can ensure data integrity and enhance the overall user experience. As Laravel continues to evolve, it's essential to stay up-to-date with the latest releases and documentation to make the most of the framework's powerful features, resulting in cleaner, more maintainable code and efficient development processes.